A great way to test an angular directive concept out outside of a project is to set up a sandbox version in jsfiddle.
Go to jsfiddle.net
Laying down the foundation for angular js code in fiddle
HTML
In the html block add:
1
| <div ng-app="myApp"></div> |

Note: ng-app=”…” can be any value you choose, I just chose “myApp”
Javascript
Then in the javascript block add:
1
| angular.module('myApp', []); |

Your module is the name you chose to use as your app name, I chose “myApp”.
Also make sure your javascript settings are set. I set mine to the following:
Language
Javascript
Frameworks & Extensions
Angular JS 1.4.8
Load Type
No wrap – in <head>

Use console to track errors
Open your console. To do this on a windows pc you press F12, and a console tab opens at the bottom (or side) of the screen. Make sure you are viewing the “console” tab.
Keep your eye on the console tab for any errors. At this point you should not see any errors, if you do, go through the steps above again. You want to proceed with no errors so it makes it easier for you to debug your directive.
Tip: You can also use console.log like normal to track manual logs while you are coding.
Click the “run” button to the top of your screen and check that no errors occur in the console tab.
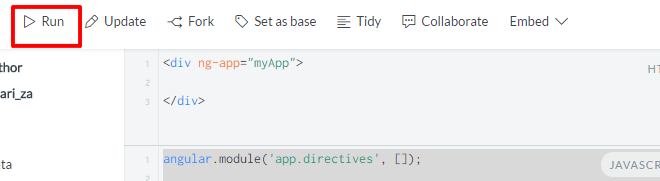
Setting up your directive
Add the directives namespace at the top of the javascript code and add the namespace to the app declaration:
1 2 3
| angular.module('directives', []);
angular.module('myApp', [<strong>'directives'</strong>]); |
You should run your code, check no errors occur and proceed.
Let’s set up a simple display directive with just a sentence to be displayed.
Simple angular js directive with a tempalte
You can create directives with html internally in the js script.
In the javascript block add the following
1 2 3 4 5 6
| angular.module('directives', []).directive('display', function(){
return {
template: 'This is just a display'
}
});
angular.module('myApp', ['directives']); |
In the html block add the following:
You can run the project now and in the bottom right you should see the sentence “This is just a display”.
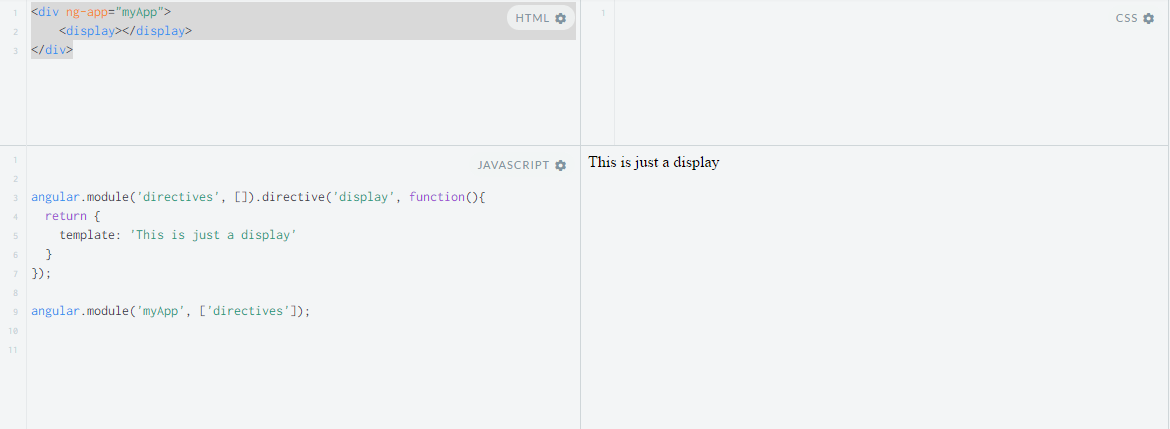
Done
That’s it. Now you can expand on your directive, look at some examples on the angular js documentation and try out more advanced directives. Add controller, link functions and template urls.
Here is the fiddle link if you would like to see it in action:
https://jsfiddle.net/cari_za/7115oy9z/1/